Introduction to Programming Using Visual Basic
Programming Using Visual Basic is a beginner-friendly programming language widely used to develop Windows applications. It is known for its intuitive graphical user interface and its focus on rapid application development. This article will provide you with a comprehensive introduction to Visual Basic programming, covering the following topics:
- Core concepts of programming
- The Visual Basic environment
- Programming basics
- User interface design
- Event-driven programming
- Data handling
Core Concepts of Programming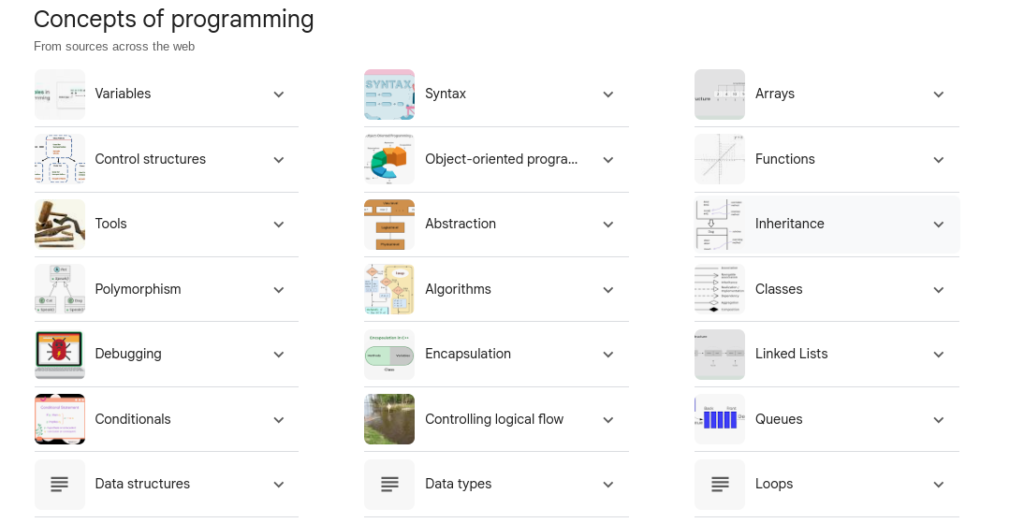
Variables
Variables are containers used to store data in a computer program. They have a name and a value, and the value can be changed during the execution of the program.
Data types
Data types define the type of data that a variable can hold. Common data types include:
- Integer: Whole numbers
- Floating-point: Decimal numbers
- String: Text
- Boolean: True or false values
Operators
Operators are symbols that perform operations on data. Common operators include:
- Arithmetic operators (+, -, *, /)
- Comparison operators (==, !=, <, >, <=, >=)
- Logical operators (&&, ||, !)
Expressions
Expressions are combinations of variables, data, and operators that evaluate a single value. For example, the expression x + y evaluates to the sum of the values of the variables x and y.
Control flow
Control flow statements control the order in which statements in a program are executed. Common control flow statements include:
- Conditional statements (if-else)
- Looping statements (for, while)
Functions
Functions are reusable blocks of code that perform specific tasks. They can be called from other program parts and return a value.
Arrays
Arrays are collections of data items of the same type. They are stored in contiguous memory locations and can be accessed using an index.
- These core concepts are fundamental to programming in any language, not just Visual Basic. By understanding these concepts, you will be well-equipped to start writing your programs.
The Visual Basic Environment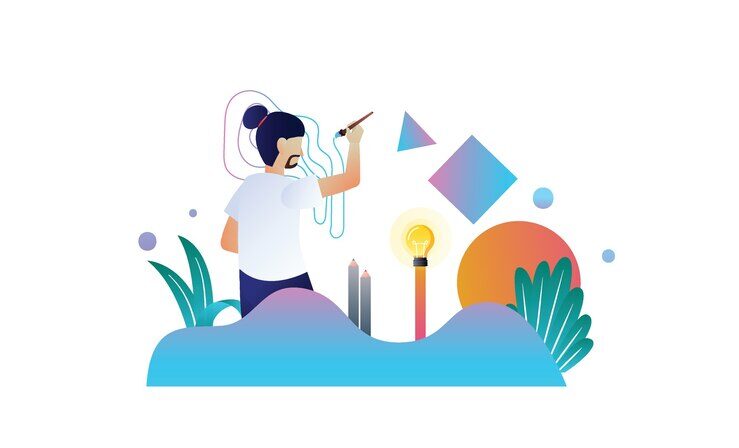
The Visual Basic environment consists of the following key components:
- Integrated Development Environment (IDE): The IDE is the graphical interface where you write and debug your Visual Basic programs. It includes various tools and features to help you develop your applications, such as a code editor, debugger, and toolbox.
- Project types: Visual Basic allows you to create different kinds of applications, including Windows forms applications, console applications, and class libraries. Each project type has its own set of features and requirements.
- Forms and controls are your applications’ graphical user interface (GUI). You can add various controls to your forms, such as buttons, labels, text boxes, and combo boxes, to create the desired user experience.
- Event handling: Visual Basic uses an event-driven programming model, which means that your code responds to events triggered by user interactions or system events. You can handle events by writing event handlers and procedures executed when a specific event occurs.
The Visual Basic environment is designed to be user-friendly and efficient, making it a popular choice for beginners and experienced developers.
Programming Basics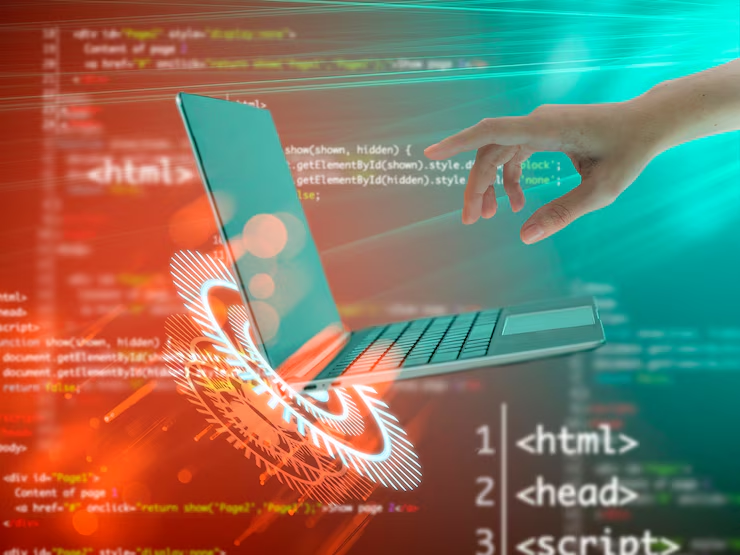
Programming basics in Visual Basic involve understanding the fundamental programming concepts, such as variables, data types, operators, expressions, control flow, functions, and arrays.
Variables
Variables are used to store data in your program. They have a name and a data type, which determines the data type the variable can hold (e.g., integer, string, boolean).
Data types
Data types define the type of data that a variable can hold. Common data types in Visual Basic include:
- Integer: Whole numbers
- String: Text
- Boolean: True or false values
- Double: Decimal numbers
Operators
Operators are used to perform operations on data. Common operators in Visual Basic include:
- Arithmetic operators (+, -, *, /)
- Comparison operators (==, !=, <, >, <=, >=)
- Logical operators (And, Or, Not)
Expressions
Expressions are combinations of variables, data, and operators that evaluate a single value. For example, the expression x + y evaluates to the sum of the values of the variables x and y.
Control flow
Control flow statements control the order in which statements in your program are executed. Common control flow statements in Visual Basic include:
- If-Elsestatements: Used to execute different blocks of code depending on whether a condition is true or false.
- Loopstatements: Used to repeat a block of code a specified number of times or until a condition is met.
Functions
Functions are reusable blocks of code that perform specific tasks. They can be called from other parts of your program and return a value.
Arrays
Arrays are used to store collections of data items of the same type. They are stored in contiguous memory locations and can be accessed using an index.
Understanding these programming basics will provide a solid foundation for developing Visual Basic applications.
User Interface Design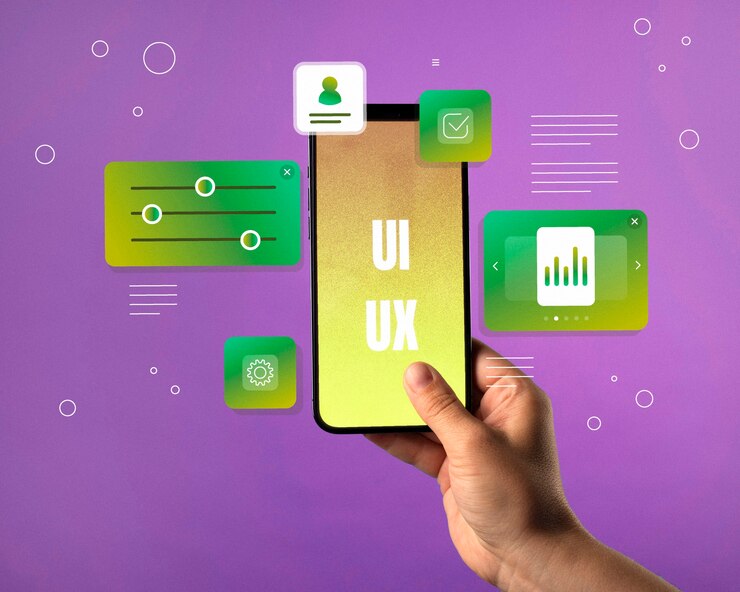
User interface (UI) design in Visual Basic involves creating the visual elements of your application that users interact with, such as forms, controls, and menus.
Forms
Forms are the graphical user interface (GUI) of your application. They contain the controls that users interact with, such as buttons, labels, text boxes, and combo boxes.
Controls
Controls are the individual elements that make up your forms. Each control has its own set of properties and methods that you can use to customize its appearance and behavior.
Menus
Menus allow users to access commands and features in your application. You can create menus using the Menu Editor in the Visual Basic IDE.
Layout
The layout of your forms and controls is essential for creating a user-friendly and visually appealing interface. You can use the Layout Manager in the Visual Basic IDE to arrange your controls and create a visually attractive design.
Event handling
Event handling is a fundamental aspect of UI design in Visual Basic. An event is triggered when a user interacts with a control, such as by clicking a button or entering text in a text box. You can handle events by writing event handlers and procedures executed when a specific event occurs.
You can create visually appealing and user-friendly applications in Visual Basic by understanding UI design concepts and techniques.
Event-Driven Programming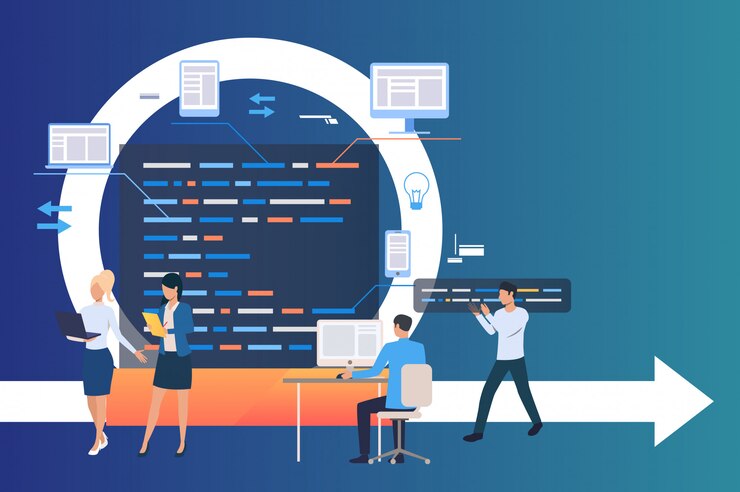
Event-driven programming is a programming paradigm in which events determine the flow of the program. Events are occurrences that happen during the execution of a program, such as user input, mouse clicks, or system events.
In Visual Basic, event-driven programming is implemented using event handlers. Event handlers are procedures that are executed when a specific event occurs. To handle an event, you can create an event handler in the Visual Basic IDE code editor.
The following steps outline how event-driven programming works in Visual Basic:
- An event occurs, such as a button click or a mouse movement.
- The event is passed to the appropriate event handler.
- The event handler executes the code that is necessary to handle the event.
- The program continues to execute.
Event-driven programming is a powerful technique for creating responsive and interactive applications. It is widely used in Visual Basic and other programming languages.
Here are some examples of how event-driven programming is used in Visual Basic:
- Button click event: When a user clicks a button, the Click event is triggered. You can write a Click event handler to perform a specific action when clicking the button, such as opening a new form or saving a file.
- Mouse move event: When the mouse moves over a control, the control’s MouseMove event is triggered. You can write a MouseMove event handler to perform a specific action when the mouse moves over the control, such as displaying a tooltip.
- Timer event: The Timer control raises the Tick event at regular intervals. You can write a Tick event handler to perform a specific action regularly, such as updating a progress bar or refreshing data from a database.
You can create responsive and interactive Visual Basic applications by understanding event-driven programming.
Data Handling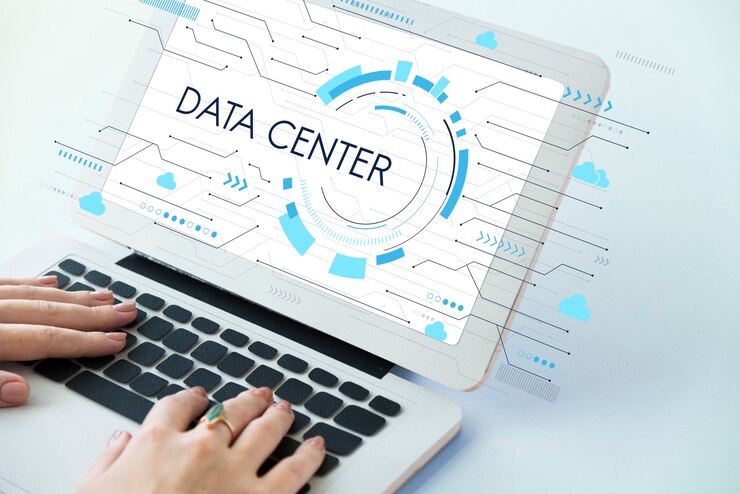
Data handling is an essential aspect of programming, and Visual Basic provides various features and techniques for working with data.
Variables
Variables are used to store data in your program. They have a name and a data type, which determines the data type the variable can hold (e.g., integer, string, boolean).
Data Types
Visual Basic supports a variety of data types, including:
- Integer: Whole numbers
- Double: Decimal numbers
- String: Text
- Boolean: True or false values
- Date: Dates and times
Arrays
Arrays are used to store collections of data items of the same type. They are stored in contiguous memory locations and can be accessed using an index.
Collections
Collections are similar to arrays but are more flexible and can store data items of different types.
Data Binding
Data binding is a technique that allows you to link data sources to controls on your forms. This makes it easy to display and update data in your application.
ADO.NET
ADO.NET is a library that provides access to data sources such as databases. It allows you to read, write, and update data in a database.
XML
XML (Extensible Markup Language) is a popular format for storing and exchanging data. Visual Basic provides support for reading and writing XML data.
Files
You can also read and write data to files using Visual Basic. This allows you to store data permanently on the user’s computer.
- By understanding data handling techniques in Visual Basic, you can effectively manage and manipulate data in your applications. This enables you to create robust and data-driven applications.
Conclusion: Programming Using Visual Basic
This article has provided you with a comprehensive overview of Programming Using Visual Basic. By understanding the core concepts, the Visual Basic environment, programming basics, user interface design, event-driven programming, and data handling, you will be well-equipped to start developing your own Windows applications using Visual Basic.